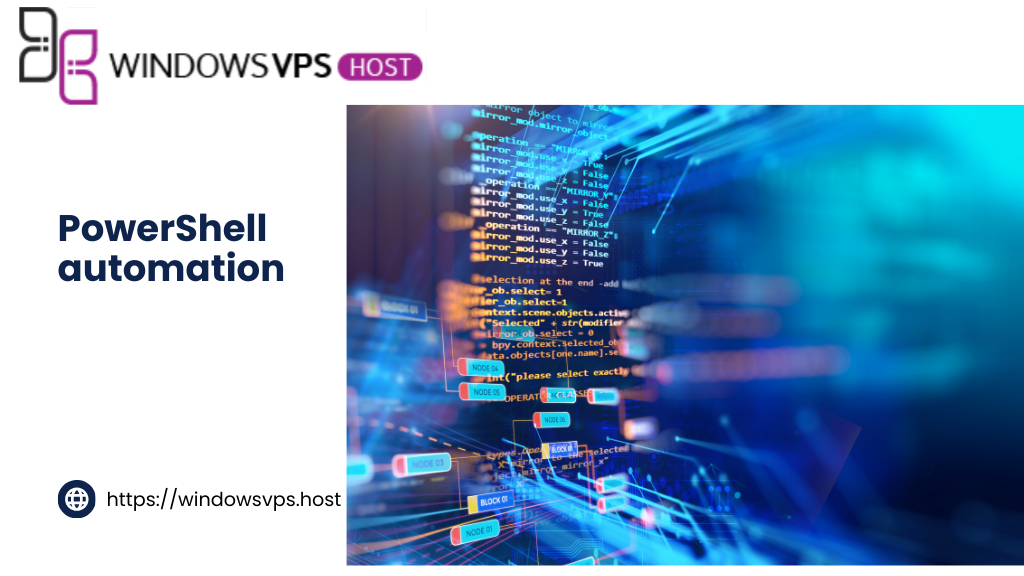
Introduction to PowerShell Scripting
Have you ever wished you could streamline the repetitive tasks you perform on your Windows VPS? With PowerShell scripting, your wish can become a reality. Whether you’re a seasoned IT professional or a curious beginner, diving into the world of PowerShell can transform the way you manage your systems. Imagine automating tasks like backups, updates, and configurations—saving you both time and effort.
This guide will walk you through the essentials of PowerShell scripting, offering you the building blocks to automate your day-to-day tasks efficiently. By the end, you’ll not only understand how PowerShell works but also how to harness its power to make your life easier. Ready to get started? Let’s dive in!
Benefits of Automating Tasks on Windows VPS
Automating tasks on your Windows VPS using PowerShell scripts can significantly increase efficiency and reduce human error. Think about the time you spend on routine maintenance, updates, and system checks. By scripting these tasks, you free up valuable time that can be redirected toward more strategic, high-value work. It’s not just about saving time though; it’s about consistency and reliability.
When you automate, every task is executed precisely the same way, every time. This eliminates the variability inherent in manual execution and makes troubleshooting much easier. For example, if a daily backup script fails, you can be quite certain the issue is in the environment, not in a deviation in the process. Furthermore, automation scales effortlessly. What might take hours to do manually can be scripted to run in minutes, regardless of the size of the workload.
Another compelling benefit is enhanced security. Human error can introduce vulnerabilities; automated scripts can adhere strictly to security policies, ensuring that sensitive tasks are conducted correctly. With PowerShell, you can script complex password resets, log and monitor unauthorized access, and more. These scripts act as your vigilant, unfaltering IT assistant, always on duty.
Setting Up Your Windows VPS for Automation
To get started with automating tasks on your Windows VPS, you first need to ensure your environment is ready for PowerShell scripting. Here are the steps you need to follow:
1. Enable PowerShell Remoting
PowerShell Remoting is pivotal for managing your VPS remotely. Open PowerShell as an administrator and run the following command:
Enable-PSRemoting -Force
This command configures your system to receive remote commands. You might also need to adjust firewall settings to allow PowerShell remoting connections.
2. Set Execution Policy
The execution policy determines the conditions under which PowerShell loads configuration files and runs scripts. To change the execution policy, use:
Set-ExecutionPolicy RemoteSigned
The RemoteSigned
policy allows scripts downloaded from the internet to run if they are signed by a trusted publisher.
3. Install PowerShell Core (Optional)
For additional features and cross-platform compatibility, you might want to install PowerShell Core. Download it from the official GitHub page and follow the installation instructions.
4. Update PowerShell Modules
Keeping your PowerShell modules up-to-date ensures you have the latest cmdlets and features. Run the following command:
Update-Module
This command updates all installed modules. You can also update individual modules if needed.
5. Verify System Requirements
Make sure your VPS meets the system requirements for running PowerShell scripts. Ensure you have the latest version of Windows Server and that your system is up-to-date with patches and updates.
With these preparatory steps completed, your Windows VPS is now set up for automation using PowerShell scripts. You’ll be well on your way to streamlining your workflow and saving time on routine tasks.
Basic PowerShell Commands You Need to Know
Before diving into complex automation tasks, it’s essential to familiarize yourself with some basic PowerShell commands. Here are a few you need to get started:
Get-Help
The Get-Help
cmdlet is your first stop when learning about any new command. It provides detailed information on how to use cmdlets, complete with examples. Simply type Get-Help <cmdlet-name>
to access the information.
Get-Command
If you’re unsure of the specific command you need, Get-Command
is incredibly useful. Running Get-Command
lists all available cmdlets, functions, aliases, and workflows. You can also search for commands related to a specific task, like so: Get-Command *service*
.
Get-Service
The Get-Service
cmdlet helps you list all the services installed on your system. You can filter to see only the running services by using: Get-Service | Where-Object {$_.Status -eq "Running"}
. This command is crucial for monitoring and managing services.
Set-ExecutionPolicy
To run scripts smoothly, you’ll often need to adjust the script execution policy. The cmdlet Set-ExecutionPolicy
allows you to change this setting. For instance: Set-ExecutionPolicy RemoteSigned
allows scripts downloaded from the internet to run if signed by a trusted publisher.
Get-Process
Want to see what processes are running on your system? Get-Process
lists them along with useful details like process ID, CPU usage, and more. You can get information about a specific process by specifying its name: Get-Process chrome
for example.
Start-Process
With Start-Process
, you can start one or more processes on your local computer. This cmdlet is handy for launching applications or scripts programmatically. For example: Start-Process notepad.exe
opens Notepad.
Stop-Process
If you need to terminate a running process, Stop-Process
is the command for the job. Specify the process by its ID or name: Stop-Process -Name "notepad"
.
Get-Item
This cmdlet retrieves an item from a specified location. It’s versatile and works with file systems, registries, and more. For example: Get-Item -Path "C:\Temp\example.txt"
retrieves the specified file.
Set-Item
With Set-Item
, you can change the value of an item. This cmdlet is useful for updating properties of files, directories, and registry keys. For example: Set-Item -Path "HKCU:\Software\MyApp" -Value "NewValue"
updates a registry key value.
Arming yourself with these basic PowerShell commands will prepare you for more advanced scripting and automation tasks. Experiment with each command, read their help files, and see how they interact with your system. The more comfortable you are with these basics, the smoother your automation journey will be.
Creating Your First PowerShell Script
Ready to dive in? Let’s get started with creating your first PowerShell script. Begin by opening your preferred text editor – Notepad, Visual Studio Code, or PowerShell Integrated Scripting Environment (ISE) will do just fine. For this example, we’ll use the ISE for its built-in support and simplicity.
1. Open PowerShell ISE:
press Windows Key + R, type powershell_ise, and hit Enter.
2. Create a New Script:
Click on File and then New to open a new script pane.
3. Write Your Script: Here’s a simple example that greets you with a “Hello, World!” message.
Write-Output "Hello, World!"
4. Save Your Script: Save the script with a .ps1 extension, such as HelloWorld.ps1.
Click File then Save As and set the file name to HelloWorld.ps1.
5. Run Your Script: You can run your script directly in the ISE by pressing F5. Alternatively, you can execute it in PowerShell:
& .\HelloWorld.ps1
If everything is set up correctly, you should see “Hello, World!” output in the console. Congratulations, you’ve written and executed your first PowerShell script!
Next, let’s spice things up with some variables and loops to make your script more dynamic. Consider a script that lists all files in a directory:
# Define the directory
$directory = "C:\YourDirectory"
# Get list of files
$files = Get-ChildItem -Path $directory
# Loop through the files and print their names
foreach ($file in $files) {
Write-Output $file.Name
}
Save the script as ListFiles.ps1 and run it the same way you did with your first script. This time, you should see a list of file names from the specified directory.
By now, you should have a basic understanding of how to create, save, and execute PowerShell scripts. The more you practice, the more comfortable you’ll become with scripting. Up next, we’ll look at how to schedule these scripts, so routine tasks can run automatically without your intervention.
Scheduling PowerShell Scripts with Task Scheduler
Scheduling your PowerShell scripts ensures they run at specific times or under certain conditions without manual intervention. To achieve this, we leverage the Task Scheduler, a built-in utility in Windows. Here’s a step-by-step guide to getting you started:
Creating a Basic Task
- Open Task Scheduler: Press Win + R, type
taskschd.msc
, and press Enter. - Navigate to ‘Create Basic Task’: In the right-hand pane, click on Create Basic Task….
- Set Task Name and Description: Give your task a meaningful name and description. Click Next.
- Trigger the Task: Choose when you want the task to start. Options include daily, weekly, monthly, or when a specific event is logged. Click Next.
- Action to Perform: Select Start a program and click Next.
- Set the Program/Script: In the Program/script field, enter
powershell.exe
. In the Add arguments (optional) field, input the path to your script, e.g.,-File "C:\Scripts\YourScript.ps1"
. - Finish the Task: Review your settings and click Finish to create the task.
Advanced Task Configuration
If you require more granular control over the task, you can configure additional settings:
- Conditions: Specify conditions for the task, such as only running if the computer is idle or connected to AC power.
- Settings: Define additional settings like what to do if the task fails or how many times to retry.
To access these options, right-click on your newly created task and choose Properties. Navigate through the Conditions and Settings tabs to fine-tune your task.
Testing Your Scheduled Task
Once your task is set up, it’s crucial to test it:
- In the Task Scheduler, locate your task under Task Scheduler Library.
- Right-click on the task and select Run.
- Check the task’s results in the History tab to ensure it executed successfully.
Scheduling PowerShell scripts with Task Scheduler can significantly streamline your workflow, ensuring that your automated tasks run smoothly and punctually. With these steps, you’ll be on your way to a more efficient Windows VPS management routine.
Automating File Management Tasks
Managing files manually can be tedious, especially when handling large volumes of data or performing repetitive tasks. PowerShell scripts come to the rescue with their robust set of cmdlets, enabling you to streamline your file management processes efficiently.
Create, Copy, Move, and Delete Files
One of the fundamental tasks in file management is handling files—creating, copying, moving, and deleting them. Let’s explore how to perform these actions using PowerShell:
- Create a File: Use the
New-Item
cmdlet to create a new file. For instance, to create a text file named “newfile.txt” in C:\Temp, you can use:New-Item -Path "C:\Temp\newfile.txt" -ItemType "file"
- Copy a File: The
Copy-Item
cmdlet is used to copy files from one location to another. For instance, to copy “newfile.txt” to D:\Backup:Copy-Item -Path "C:\Temp\newfile.txt" -Destination "D:\Backup\newfile.txt"
- Move a File: Use the
Move-Item
cmdlet to move files. For example, to move “newfile.txt” from C:\Temp to D:\Archive:Move-Item -Path "C:\Temp\newfile.txt" -Destination "D:\Archive\newfile.txt"
- Delete a File: The
Remove-Item
cmdlet helps you delete files. To delete “newfile.txt” from D:\Archive:Remove-Item -Path "D:\Archive\newfile.txt"
Automate File Organization
Organizing files into appropriate folders can greatly enhance your workflow efficiency. Here’s how to automate this using PowerShell:
Suppose you need to organize files by their extensions. You can script this process as follows:
$sourceDir = "C:\Downloads"
$targetDir = "C:\SortedFiles"
# Get all files in the source directory
$files = Get-ChildItem -Path $sourceDir
foreach ($file in $files) {
$extension = $file.Extension.TrimStart('.')
$destDir = "$targetDir\$extension"
# Create destination directory if it doesn't exist
if (-not (Test-Path -Path $destDir)) {
New-Item -ItemType Directory -Path $destDir
}
# Move file to the destination directory
Move-Item -Path $file.FullName -Destination $destDir
}
Monitor and Manage Disk Space
Ensuring sufficient disk space is crucial for system performance and stability. PowerShell scripts can help you monitor and manage disk usage:
Get-PSDrive -PSProvider FileSystem |
Select-Object Name, @{Name="Used(GB)";Expression={[math]::round($_.Used/1GB,2)}}, @{Name="Free(GB)";Expression={[math]::round($_.Free/1GB,2)}}
This script retrieves the used and free disk space for each drive, making it easier to keep an eye on your storage capacity.
By incorporating these PowerShell scripts into your task automation, file management on your Windows VPS becomes a breeze, freeing up your time for more complex and creative tasks.
Automating System Maintenance Tasks
Keeping your system in top shape is crucial. Luckily, PowerShell offers a plethora of commands and script capabilities to automate everyday maintenance tasks effortlessly. From clearing temporary files to defragmenting the disk, you can set up scripts to run these tasks regularly, ensuring your Windows VPS stays efficient and operates smoothly.
Clearing Temporary Files
Temporary files can pile up quickly, eating away at valuable disk space. PowerShell can help you automate the process of deleting these unnecessary files.
Here’s a simple script to clear temporary files:
Remove-Item -Path "C:\Windows\Temp\*" -Recurse -Force
Remove-Item -Path "$env:TEMP\*" -Recurse -Force
Save this script to a .ps1
file and schedule it to run at regular intervals using Task Scheduler. This ensures your system remains clutter-free without manual intervention.
Disk Defragmentation
Defragmenting your disk can enhance system performance by organizing fragmented files. PowerShell simplifies this task with its command integration.
To defragment a drive, use the following command:
Optimize-Volume -DriveLetter C -Defrag -Verbose
This command runs a defragmentation process on your C: drive. You can add it to a script and schedule it for routine execution.
Updating System Software
Regular software updates are pivotal for system security and performance. While Windows Update handles most system updates, third-party software might need additional attention.
PowerShell can automate installation and updates for various applications. For instance, if you’re using Chocolatey, a popular package manager, you can create scripts to update your software:
choco upgrade all -y
Incorporate this line into a PowerShell script and schedule it to ensure that all your software remains up-to-date with minimal effort.
System Reboot
Automated system reboots can help clear memory and apply updates. With PowerShell, scheduling a system reboot is straightforward:
Restart-Computer -Force
Beware of scheduling reboots at critical times. Ensure they occur during off-peak periods to prevent disruption.
Backup and Restore
Creating regular backups is a fundamental aspect of system maintenance. PowerShell can facilitate both file and system state backups effortlessly.
Here’s a simple script to backup files:
Copy-Item -Path "C:\ImportantFiles" -Destination "D:\Backup" -Recurse
This command copies all files from C:\ImportantFiles
to D:\Backup
. Regularly scheduling this script can prevent data loss and ensure recovery options are always up-to-date.
By leveraging PowerShell for these system maintenance tasks, you can ensure your Windows VPS remains reliable and efficient, with minimal manual intervention. Schedule these scripts using Task Scheduler to automate and optimize your server maintenance routines continuously.
Automating Software Installation and Updates
Installing and updating software on a Windows VPS can be a tedious task if done manually. Fortunately, with PowerShell, you can automate these processes, saving you time and effort. In this section, we’ll explore how to use PowerShell scripts to handle software installation and updates effectively.
Installing Software with PowerShell
PowerShell provides several cmdlets that make software installation straightforward. You can leverage tools like Chocolatey
, a package manager for Windows, to streamline this process.
First, you’ll need to install Chocolatey on your system. Open a PowerShell window with administrative privileges and run the following command:
Set-ExecutionPolicy Bypass -Scope Process -Force; `
[System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol `
-bor 3072; iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1'))
Once installed, you can leverage Chocolatey to install software packages. For example, to install Google Chrome, run:
choco install googlechrome -y
Updating Software with PowerShell
Keeping your software up to date is vital for security and performance. PowerShell can also automate this process using Chocolatey. To update all installed packages, simply execute:
choco upgrade all -y
This command checks for updates for all installed Chocolatey packages and applies them accordingly.
Using PowerShell Modules for Installation and Updates
Besides Chocolatey, PowerShell modules like PSWindowsUpdate
can also help manage updates, particularly for Windows components. First, you need to install the module:
Install-Module -Name PSWindowsUpdate -Force
After installation, you can use cmdlets from this module to manage Windows updates:
Get-WindowsUpdate
This command lists available updates. To install them, run:
Install-WindowsUpdate -AcceptAll -AutoReboot
Scheduling Software Updates
For convenience, you can schedule these tasks to run at specific intervals using Task Scheduler. Refer back to the section “Scheduling PowerShell Scripts with Task Scheduler” to set this up, automating your software maintenance tasks entirely.
Automating software installation and updates with PowerShell ensures your system remains up-to-date without manual intervention, enhancing both efficiency and security.
Using PowerShell to Monitor Server Health
Monitoring the health of your server is crucial to ensure its optimal performance and prevent potential issues. PowerShell provides a powerful set of cmdlets and scripts that can help you keep an eye on various aspects of your server’s health. Let’s dive into some of the key areas you can monitor using PowerShell.
Checking CPU Usage
High CPU usage can slow down your server and impact the performance of applications. You can use the Get-Counter
cmdlet to retrieve CPU usage data. Here’s an example:
Get-Counter -Counter "\Processor(_Total)\% Processor Time"
This command will return the total percentage of processor usage. To monitor CPU usage over time, you can schedule this script to run at regular intervals and log the results.
Monitoring Memory Usage
Memory usage is another critical metric to keep an eye on. The Get-Process
cmdlet can provide detailed information about memory consumption:
Get-Process | Select-Object Name, WS
This script will list the working set (memory usage) for each process. For a more summarized view, you can use the Get-Counter
cmdlet:
Get-Counter -Counter "\Memory\Available MBytes"
This command returns the available memory in megabytes.
Disk Utilization
Disk space is finite, and running out of it can cause critical failures. Use the Get-PSDrive
cmdlet to check disk utilization:
Get-PSDrive -PSProvider FileSystem
This script lists all file system drives and their used and free space. For more detailed monitoring, you can integrate this command into a larger script that tracks disk space usage over time.
Network Utilization
Network performance can affect everything from web applications to file transfers. Monitor network utilization using the Get-NetAdapterStatistics
cmdlet:
Get-NetAdapterStatistics
This command provides detailed statistics about the network adapters, including bytes sent and received. You can track these values over time to identify bottlenecks or unusual activity.
Service Health
Critical services should always be running smoothly. Use the Get-Service
cmdlet to check the status of important services:
Get-Service -Name "YourServiceName"
This command returns the status of the specified service. You can create a script to check multiple services and send alerts if any service is not running.
By leveraging these PowerShell commands, you can set up a comprehensive monitoring system for your Windows VPS. Regular monitoring will help you identify and resolve issues before they escalate, ensuring your server runs smoothly and efficiently.
Troubleshooting Common PowerShell Issues
Even seasoned PowerShell users can run into issues that can stall their automation tasks. Here, we’ll walk you through some common problems and provide troubleshooting tips to help you keep your scripts running smoothly.
No Execution Policy Set
If you encounter an error stating that the execution of scripts is disabled on your system, this is likely due to the execution policy settings. You can verify the current execution policy by running:
Get-ExecutionPolicy
To resolve this, you might need to set the policy to allow script execution. For instance, you can set it to ‘RemoteSigned’ using:
Set-ExecutionPolicy RemoteSigned
Ensure you run this command with administrative privileges.
Untrusted Script Warning
When running scripts downloaded from the internet, you may receive a warning that the script is untrusted. To resolve this, you can unblock the script by right-clicking on the file, selecting ‘Properties’, and clicking ‘Unblock’. Alternatively, you can use PowerShell to unblock the file:
Unblock-File -Path "C:\path\to\your\script.ps1"
Insufficient Permissions
Lack of administrative privileges can prevent scripts from executing successfully. Ensure you’re running PowerShell as an Administrator by right-clicking on the PowerShell icon and selecting ‘Run as Administrator’. You can also check the permissions on specific files or folders to make sure your script has the necessary access rights.
Module Not Found
If your script requires specific PowerShell modules, and they aren’t available on your system, you’ll encounter errors. You can install the required modules using the following command:
Install-Module -Name ModuleName
Make sure you replace ‘ModuleName’ with the actual name of the module you need.
Command Not Recognized
Sometimes, the command or cmdlet you’re trying to use might not be available. This can happen if you’re using an older version of PowerShell. To fix this, ensure your PowerShell is updated to the latest version. You can update PowerShell by downloading the latest version from the official PowerShell GitHub repository.
Script Execution Unexpectedly Terminated
If your script stops unexpectedly, it might be due to unhandled exceptions or errors within the script. PowerShell
By addressing these common issues, you can troubleshoot effectively and ensure your automation tasks on Windows VPS run without hitches. Being proactive in handling these issues will save you time and make your PowerShell scripts more reliable.
provides robust error handling through Try, Catch, and Finally blocks. Implementing these in your scripts can help you manage unexpected terminations and allow your script to exit gracefully.
Try {
# Your code here
}
Catch {
Write-Error "An error occurred: $_"
}
Finally {
# Cleanup code here
}
Advanced PowerShell Techniques for Automation
Once you’ve mastered the basics of PowerShell scripting, it’s time to delve into some advanced techniques that can significantly enhance your automation capabilities. These advanced techniques will help you fine-tune your scripts for efficiency, reliability, and scalability. Let’s explore some powerful features and best practices to take your PowerShell skills to the next level.
Leveraging PowerShell Functions
Creating reusable functions is a cornerstone of efficient scripting. Functions allow you to encapsulate repetitive tasks into single units, which you can call multiple times within your script. This not only makes your code cleaner but also easier to maintain.
function Get-UserHomeDirectory {
param (
[string]$username
)
Get-ADUser -Identity $username | Select-Object -ExpandProperty HomeDirectory
}
In the example above, Get-UserHomeDirectory
is a custom function that retrieves the home directory of an Active Directory user. You can call this function with different usernames throughout your script without rewriting the logic.
Error Handling with Try/Catch
Robust error handling is critical for creating reliable scripts. PowerShell’s try
and catch
blocks allow you to gracefully handle errors and take corrective actions.
try {
# Attempt to open a file
$fileContent = Get-Content -Path "C:\nonexistentfile.txt"
}
catch {
Write-Error "An error occurred: $_"
# Perform additional error handling
}
By using error handling, you ensure your scripts can manage unexpected issues without crashing, providing a smoother user experience.
Automating with PowerShell Workflows
PowerShell workflows enable you to perform long-running tasks and survive disruptions like network failures or system reboots. Workflows are particularly useful for tasks such as deploying software, configuring servers, or running complex sequences.
workflow DeployApplication {
Parallel {
Install-Package -Name "App1"
Install-Package -Name "App2"
}
Restart-Computer -Wait
InlineScript {
Write-Output "Deployment complete."
}
}
With workflows
Best Practices for Writing PowerShell Scripts
Writing clean, efficient, and maintainable PowerShell scripts can save you a lot of headaches down the road. By adopting best practices, you ensure your scripts are reliable and easier to debug or modify when future requirements arise. Here are some best practices to consider:
1. Use Consistent Naming Conventions
Adopt a clear naming convention for your scripts, functions, variables, and parameters. For example, use camelCase for variables ($myVariable
) and PascalCase for functions (Get-MyFunction
). Consistency in naming not only makes your code more readable but also helps others understand your scripts more quickly.
2. Comment Your Code
Commenting is essential for providing context and explanations for your scripts. Use #
to add comments in your code. Effective comments can describe the purpose of your script, explain complex logic, or specify parameters and outputs.
# This script checks the service status
$service = Get-Service -Name 'W32Time'
if ($service.Status -ne 'Running') {
Start-Service -Name 'W32Time'
}
3. Modularize Code with Functions
Break down your script into smaller, reusable functions. This modular approach makes your script easier to test, debug, and maintain. Each function should perform a single, well-defined task.
function Start-ServiceIfStopped {
param (
[string]$serviceName
)
$service = Get-Service -Name $serviceName
if ($service.Status -ne 'Running') {
Start-Service -Name $serviceName
}
}
4. Use Error Handling
Incorporate error handling using try
, catch
, and finally
blocks. This practice helps you manage and respond to errors gracefully, improving the robustness of your scripts.
try {
# service the
Attempt to start Start-Service -Name 'W32Time'
} catch {
Write-Host "Failed to start the service: $_"
} finally {
Write-Host "Service check complete."
}
5. Validate Input
Ensure that your script validates all user inputs using parameters and data type validation. This helps prevent unexpected errors and improves the script’s reliability.
param (
[ValidateNotNullOrEmpty()]
[string]$serviceName
)
if (-not (Get-Service -Name $serviceName -ErrorAction SilentlyContinue)) {
throw "Service not found: $serviceName"
}
6. Leverage PowerShell Modules
Use and create PowerShell modules to package and distribute your scripts. Modules allow you to organize related commands and functions and make it easy to share your work with others.
New-ModuleManifest -Path "$env:PSModulePath\MyModule.psd1" `
-Author "Your Name" `
-Description "My custom module" `
-FunctionsToExport "*"
7. Schedule Regular Reviews and Refactoring
Periodically review and refactor your PowerShell scripts. As you gain more experience and as requirements evolve, revisiting your script can enhance its performance, readability, and robustness.
By implementing these best practices, you’ll create PowerShell scripts that are efficient, maintainable, and a joy to work with.
Resources for Learning More About PowerShell
If you’re eager to dive deeper into the world of PowerShell and hone your automation skills, there are numerous resources available online and in print. Here’s a curated list to get you started on your learning journey:
- Microsoft PowerShell Documentation: The official documentation is a comprehensive resource that covers everything from basic commands to advanced scripting techniques.
- PowerShell.org: This community-driven resource offers forums, blog posts, and regular events like PowerShell summits and webinars.
- Reddit PowerShell Community: An active community where you can ask questions, share scripts, and get advice from seasoned PowerShell users.
- Pluralsight PowerShell Courses: These video courses are great for visual learners and cover a wide range of topics, from beginner to advanced levels.
- Learn Windows PowerShell in a Month of Lunches” by Don Jones and Jeffrey Hicks: A highly recommended book that breaks down learning PowerShell into manageable sessions, perfect for a busy schedule.
- PowerShell GitHub Repository: Explore the open-source development of PowerShell, contribute to the community, or simply see how the magic happens behind the scenes.
- YouTube PowerShell Channel: Watch tutorials, live demos, and expert discussions about PowerShell, hosted by industry veterans.
- SS64 PowerShell Reference: A quick reference guide for PowerShell commands with concise descriptions and examples – perfect for quick lookups.
- PowerShell eBooks by DevOps Collective: Download free eBooks on various PowerShell topics, including scripting best practices, security, and cloud automation.
These resources should equip you with the knowledge and tools to not only master PowerShell but also to leverage it to its full potential on your Windows VPS. Happy scripting!